Arrow
Arrow is a functional programming library, for which Akkurate provides an integration to convert its validation results to Arrow's typed errors.
This article will show you what conversions are possible and how they can help you bridge the gap between Akkurate and Arrow.
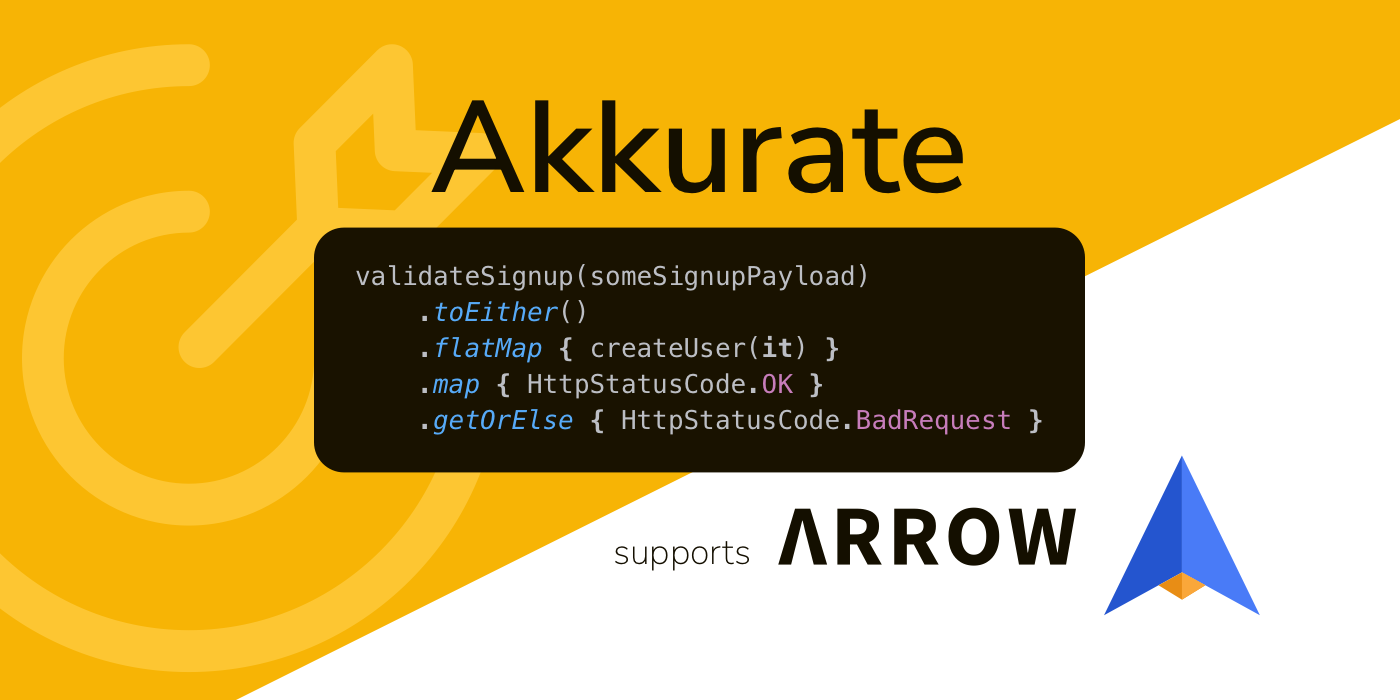
Installation
Before using Akkurate with Arrow, you need to add the following dependency to your Gradle script:
Install in a single-platform project
Install in a multiplatform project
Convert to the Either type
By calling toEither, you can convert the validation result to an Either
.
Take the following validator:
You obtain a validation result by calling the validateBook()
function:
Then, you can convert it by calling the toEither()
extension function:
On a successful validation, you can read the validated value; on a failed one, you can list all the constraint violations:
However, converting the validation result to an Either
type doesn't bring much if you only use when
expressions. Arrow is a functional library after all, so you should probably take advantage of it:
Bind to Raise computation
When working with Arrow's functional programming paradigms, the bind function is crucial in handling validations seamlessly, especially when dealing with multiple Either
types. This is particularly useful when you're already inside a Raise computation.
Let's consider an example where we have multiple data classes to validate:
In a typical scenario, you might want to validate a Book
and an Author
within the same context. Without bind, you would convert each validation result to an Either
and handle them separately, which can be cumbersome:
However, using bind
allows you to compute over the happy path by automatically handling errors and focusing on successful outcomes. When a validation fails, bind
short-circuits the computation, eliminating the need for explicit error checking and handling within the either
block.