Ktor Client
Ktor Client is a framework for building asynchronous client-side applications. Akkurate provides an integration to automatically validate downloaded data.
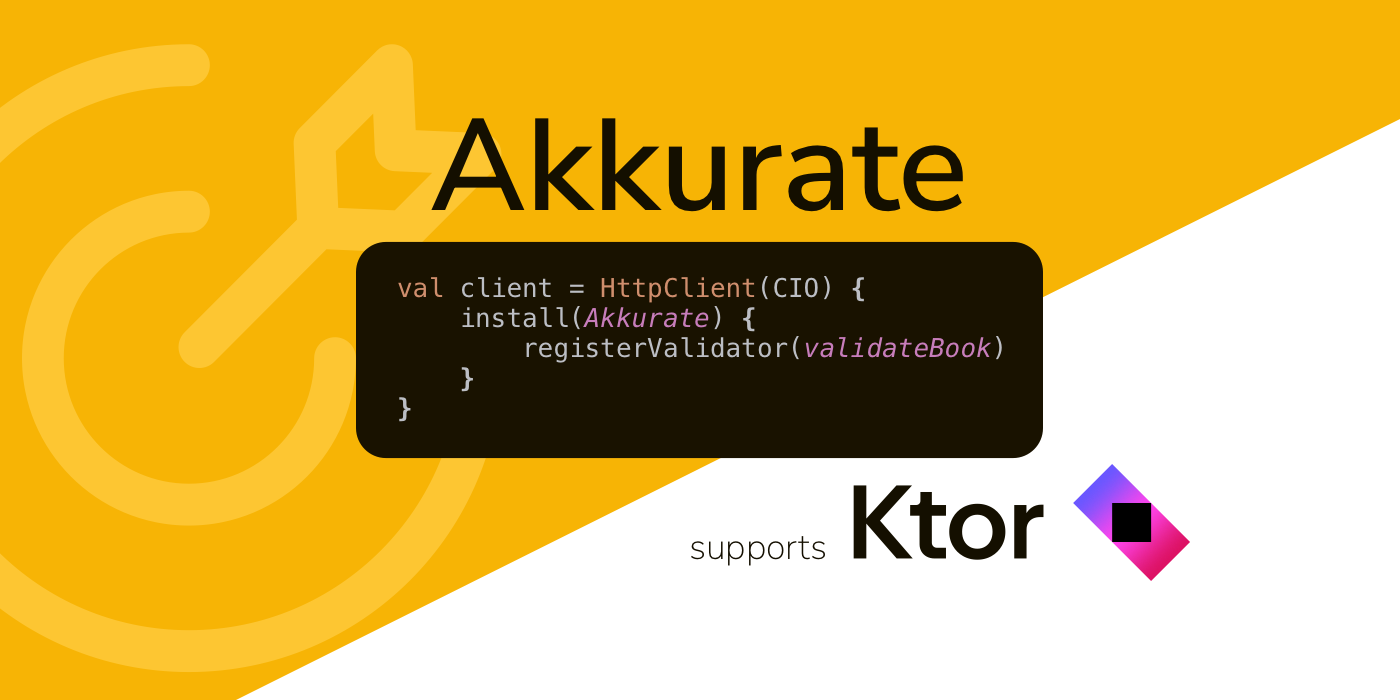
Installation
Before using Akkurate with Ktor, you need to add the following dependency to your Gradle script:
Add the Akkurate plugin for Ktor
implementation("dev.nesk.akkurate:akkurate-ktor-client:0.11.0")
Validate on deserialization
We want to retrieve the latest releases of Akkurate, using GitHub's API:
@Validate
@Serializable
data class Release(val name: String)
suspend fun main() {
val client = HttpClient(CIO) {
install(ContentNegotiation) {
json(Json { ignoreUnknownKeys = true })
}
}
val apiUrl = "https://api.github.com/repos/nesk/akkurate/releases"
val response: HttpResponse = client.get(apiUrl)
val data: List<Release> = response.body()
println(data)
}
[
Release(name=Akkurate 0.10.0),
Release(name=Akkurate 0.9.1),
Release(name=Akkurate 0.9.0),
...
]
Although this API is probably well maintained, we fear potential issues on the API side, like bugs or breaking changes. To protect ourselves, we want to validate the response body:
val validateReleaseList = Validator<List<Release>> {
each {
name.isNotEmpty()
}
}
And we register this validator in our client:
install(Akkurate) {
registerValidator(validateReleaseList)
}
Now, when deserializing the response body, the Release
instances are automatically validated, and an exception is thrown when invalid:
try {
val data: List<Release> = response.body()
println(data)
} catch (exception: ValidationResult.Exception) {
System.err.println("Invalid response: ${exception.violations}")
}
Last modified: 27 January 2025